If you do misspell in the class name, the class won’t get imported properly, and the compiler won’t be able to find the class. These names are case sensitive and you must be specifically careful with them, or the class simply won’t get recognized by the compiler. Questions: I recently started Android Studio IDE to make my android apps. I find shortkey to remove unused import in Android Studio is not working (Ctrl+Shift+O) What is shortcut key to do same in Android Studio? Answers: Simple, right click on your project in Android Studio, then click on the Optimize Imports that should work. It’s not a problem.It’s just a reminder from IDE that you have not use the method,So you can use,comment or remove the method.
I’ve found an unused string resource, like:
However it is in tens of files, of different languages, in different strings.xml
files in values
, values-af
, values-be
, etc folders.
I must have missed something not to know any way to do this in Android Studio other than modifying it by hand one by one.
tl;dr How to delete an unused string resource for all configurations?
Answers:
It is now possible inside Android Studio.
After Lint checks you see an option on the right Remove All Unused Resources!
To Delete a single string resource across all locale files, you can use the “Translation Editor”.
1. Right click on the res directory to open the translation editor.
2. Select “Show All Keys” selector, and choose “Filter by Text”. Supply the name of the resource that you want to delete.
3. Select the resource, and click on the “-” button
Answers:
To identify all unused resources:
- Open Menu > Analyze > Run Inspection by Name…
- Select “Unused Resources”
- Make sure Whole project is checked, then press OK.
- Look through the list. You can always rerun the Inspection with the Rerun button.
There is no really easy way in Android Studio (v 1.0) to remove a resource string for all locales. However, you can search and replace in files. Fortunately, the translation files use only a single line in most cases so this works pretty well.
In Android Studio:
- Start with no changes pending in your source control (makes it way easier to check and back out if this goes wrong).
- Open Menu > Edit > Find > Replace in Path…
- In Text to find:
.*name='obsoletestring'.*n
- In Replace with: (empty)
- Check Regular expression
- Press the Find button.
- Inspect the results. If okay, press the “All files” button.
- You will probably have to remove the comment in res/values/strings.xml manually.
- Make sure your project still builds.
- Take a look at the diff of your project in your source control to ensure the change is really what you intended…
Answers:
Until IDE support comes along, something along these lines will work:
Answers:
In Android Studio 2.3 it’s possible to remove all unused resources.
- Open any *.xml in your res/values/ directory
- Right click on any item’s name
Refactor -> Remove Unused Resources..
Answers:
Unfortunately, You have to do it manually.
Check this answer to understand what exactly should you do to get rid of them using Eclipse
If you are using Android Studio
find them in the whole application and also remove manually . Check this answer
Answers:
- Menu -> Analyze -> Run Inspection by Name -> Unused resources
From the results select all string resources that are unused.
- Right-click highlighted rows and choose “Suppress with @SuppressLint(Java) or tools:ignore(XML)”. This will add the attribute tools:ignore to all strings in all string files.
Menu -> Find -> Replace in Path
Caused by: java.sql.SQLSyntaxErrorException: ORA-00900: invalid SQL statement In order to describe the use cases and diagnose the problem, I have reduced the complexity of the SQL statement in the description below. Use the Jasper Studio “Dataset and Query Dialog Box” to run the report with the following SQL statement. This solution worked for most cases except that if sql script contains create or replace trigger it fails with java.sql.SQLSyntaxErrorException: ORA-00900: invalid SQL statement. I have also specified snippet of sql script which fails. Please note that if I run same script through SQL Developer, it runs fine. Following is the Java. 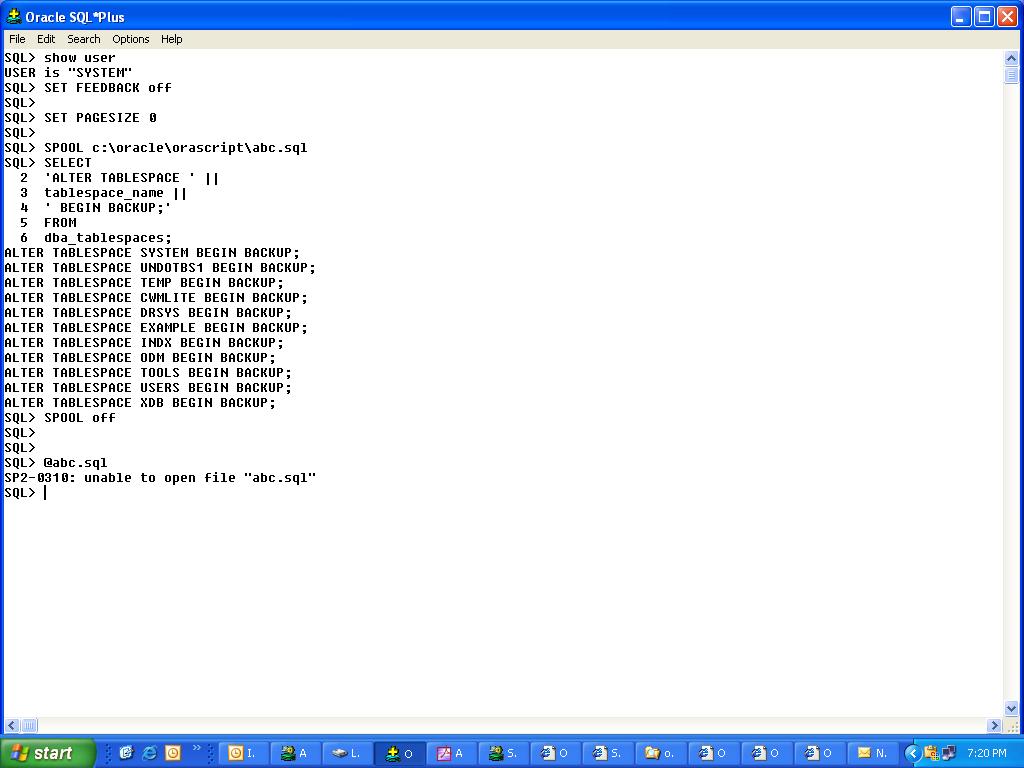
- Text to find: ^.*?tools:ignore=”UnusedResources”.*?n
- Tick regular expression box
- Use Scope: Custom
- Open custom scope editor and add pattern: file[app]:src/main/res//strings.xml
- Find
- Etc.
Answers:
In fact, Android Lint should report about the unused resources, but you can also try with this nice plugin.
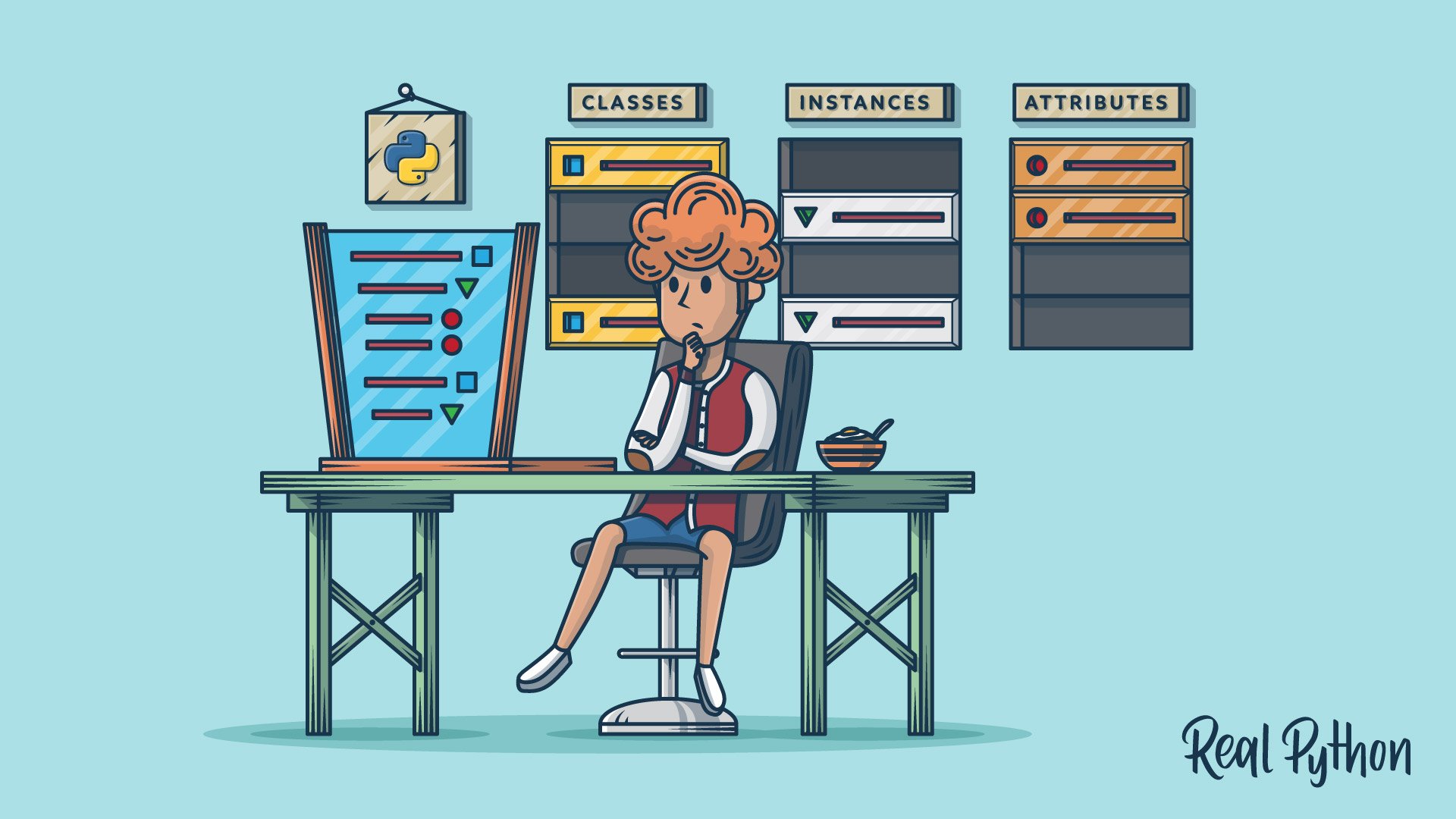
Answers:
Beware that the REMOVE UNUSED RESOURCES command cannot recognize a programmatically accessed resource as a used resource (such as getIdentifier(.) etc.).
So, if you do access resources that way, it is highly risky to use that command!!
Tags: android, sed, string
Android Studio Find Unused Classes For Beginners
Tips > Android Lint > Suppressing Lint Warnings
This page is obsolete. Redirecting to https://developer.android.com/studio/write/lint.html#config
While Lint can often find real errors that must be fixed, it also flags issues that may or may not be a problem depending on the context. If you've manually verified that an issue is not a problem, you may want to mark the issue as verified such that lint does not keep pointing it out. In SourceYou can suppress lint issues directly in the source code. This has the advantage that you filter only this specific occurrence of the lint issue (that you've presumably manually verified), not all occurrences of a particular issue type, or all occurrences in a file.With AnnotationsIn .java files, you can suppress issues with the @SuppressLint annotations. You supply the lint issue id as the argument to the annotations. For example public void onGlobalLayout() {
You can also suppress more than one issue using a comma separated list:
In both Android Studio and Eclipse you can easily suppress lint issues directly without having to write the annotation yourself. Just invoke the quickfix for the warning, and one of the options will be to ignore the issue with an annotation; this will annotate either the local declaration, or the method, or the class, or the field, depending on the location of the error. (Some screenshots of this in action in Eclipse is shown here.) In .xml files, you suppress issues with the special tools:ignore attribute instead. <FrameLayout xmlns:android='http://schemas.android.com/apk/res/android' xmlns:tools='http://schemas.android.com/tools'>
<LinearLayout
..
Tip: You can supply a comma separated list of issue id's, or the special value ' all ' to indicate all issues. In Source: With CommentsIn recent versions of lint, you can also use a special line comment on the line above the error to suppress a warning. In .java and .gradle files, use a comment line this (where again the issue id can be a comma separated list of id's). You can prefix the id with 'AndroidLint', which is allowed for consistency with the way IntelliJ disables inspections in comments.) String path = '/sdcard/legacy.txt';
In XML files, the comment should look like this: (Again, you can supply a comma separated list of issue ids instead of a single id.) In Build File: With Gradle ConfigurationIn Gradle files, you can specify a lintOptions configuration where you can disable id's, enable id's, as well as change the severity of issue id's (including to 'ignore' to disable them). From Command LineThe lint command has three commands for controlling which checks are performed: --enable , --disable and --check (see the overview document for more details on these flags). However, these must be specified each time you run lint. This is similar to the lintOptions for Gradle above. To persistently configure which rules are run, you can create a file named lint.xml in the root directory of your project (next to the manifest file). Lint will automatically look at this file and use it to ignore warnings. Gradle, Android Studio and Eclipse will also use this configuration file if present. Note that when you use Eclipse to suppress errors, it automatically creates this file for you, so you can use Eclipse to ignore warnings and then check in the resulting configuration file such that for example build server lint runs will ignore warnings you've manually verified. Here's a sample lint.xml file (the comments are obviously not needed; I've added them here to explain what each line does) <lint>
<issue severity='ignore' />
<!-- Ignore the ObsoleteLayoutParam issue in the given files -->
<ignore path='res/layout/activation.xml' />
<ignore path='res/layout-xlarge/activation.xml' />
<!-- Ignore the UselessLeaf issue in the given file -->
<!-- Change the severity of hardcoded strings to 'error' -->
</lint>
In recent versions of lint (Gradle plugin 0.9+, SDK Tools 27+, Android Studio 0.5+) you can use a globbing pattern for the path: <ignore path='res/**/activation.xml' />
You can also specify a full regular expression, by using a regexp attribute instead of path : You can also use 'all ' as the issue id if you want to apply settings for all issues. Epson l220 resetter online. Finally, as of Android Studio 0.9 and Gradle plugin 0.14.+ you can use a regular expression to match the error message as well: <ignore regexp='Invalid package reference in library; not included in Android: java.* Referenced from ' />
Issue Id'sThe issue identifiers used to suppress issues as shown above (IconMissingDensityFolder, ObsoleteLayoutParam etc) are the issue ids, which are included in the error output from the lint command: Warning: The resource R.drawable.robot appears to be unused [UnusedResources]
You can also look up the lint ids with the lint --show command (for full details) or lint --list (for a brief summary): ..
'ContentDescription': Ensures that image widgets provide a contentDescription
'DuplicateIncludedIds': Checks for duplicate ids across layouts that are
'DuplicateIds': Checks for duplicate ids within a single layout
'StateListReachable': Looks for unreachable states in a <selector>
'InefficientWeight': Looks for inefficient weight declarations in
'NestedWeights': Looks for nested layout weights, which are costly
This lint configuration file apply to the current project, as well as any library projects included into this project. If you want to also have a 'global' configuration used for all projects, you can create an additional global file and then invoke lint with the --config flag: --config <filename> Use the given configuration file to determine whether
issues are enabled or disabled. If a project contains
a lint.xml file, then this config file will be used
From Android StudioYou can configure lint severities from the global inspections menu. However, these will only be used inside Studio itself. If you want the Gradle command line build and other users to see your altered severities, use a lint.xml file or a Gradle lintOptions configuration instead. To suppress an error, open the issue in the editor and run the quickfix/intentions action (Ctrl/Cmd F1) and select Suppress which will use annotations, attributes or comments to disable the issue. From EclipseIf you're using the Eclipse Lint plugin, you can suppress errors directly from the Lint Window. There are three separate ignore levels: - Always ignore. This completely suppresses the given warning. Use this for warnings you never want flagged.
- Ignore in project. This will ignore this warning in the current project (and any library projects referenced from this project).
- Ignore in file. This will ignore this warning anywhere in the current file, but not in any other files.
- (Ignore warnings within a class, method or even for a specific variable declaration, using annotations or attributes: See this document for more information.)
You can also access these from the quickfix menu in XML editors, and the first two from the Global Options dialog and from the Project Options dialog. Project and file specific warnings are written into lint.xml files into the projects, so command line and build server lint runs will pick these up. |
|